1. Word Search in Grid 
Given an m x n grid of characters board and a string word, return true if word exists in the grid.
The word can be constructed from letters of sequentially adjacent cells, where adjacent cells are horizontally or vertically neighboring. The same letter cell may not be used more than once.
Example 1:
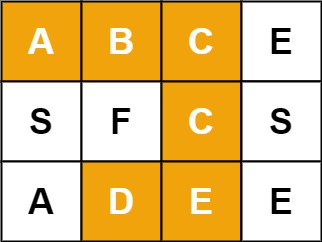
Input: board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCCED" Output: true
Example 2:
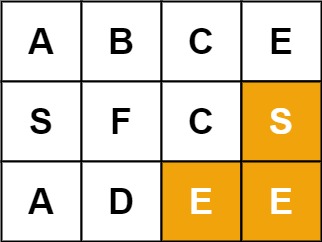
Input: board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "SEE" Output: true
Example 3:
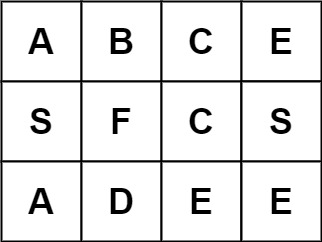
Input: board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCB" Output: false
Constraints:
- m == board.length
- n = board[i].length
- 1 <= m, n <= 6
- 1 <= word.length <= 15
- board and word consists of only lowercase and uppercase English letters.
Follow up: Could you use search pruning to make your solution faster with a larger board?
2. Case Study on Ranking
Discuss a case study related to ranking systems, including the interaction with the interviewer in a system design context.
3. System Design for 3rd Party Price Tracker for Wayfair
Design a system for a 3rd party price tracker for Wayfair. Users should be able to enter the URL of a product on Wayfair, the price they are willing to pay, and their email. The system should send an email notification when the price of the item equals or goes below the user's desired price. Assume we have 100k active users.
4. Design a System for Searching Restaurant Reviews
Given 100M restaurant reviews, design a system for searching the reviews by keywords. The system should be able to return all reviews containing all the terms in a query. Make additional assumptions as needed and state them explicitly.
5. Break a String into Words
Given text, a non-empty string, and glossary containing a list of non-empty words, add spaces in text to construct a sentence where each word is a valid dictionary word in glossary. Return all such possible sentences. For example, given the input text = 'northamericansalesdlgroup' and glossary = ['north', 'america', 'american', 'sale', 'sales', 'dl', 'group'], provide the output.