1. Snake exit
We have a two-dimensional board game involving snakes. The board has two types of squares on it: +’s represent impassable squares where snakes cannot go, and 0’s represent squares through which snakes can move.
Snakes may move in any of four directions - up, down, left, or right - one square at a time, but they will never return to a square that they’ve already visited. If a snake enters the board on an edge square, we want to catch it at a different exit square on the board’s edge.
The snake is familiar with the board and will take the route to the nearest reachable exit, in terms of the number of squares it has to move through to get there.
Write a function that takes a rectangular board with only +’s and 0’s, along with a starting point on the edge of the board (given row first, then column), and returns the coordinates of the nearest exit to which it can travel.
If multiple exits are equally close, give the one with the lowest numerical value for the row. If there is still a tie, give the one of those with the lowest numerical value for the column.
If there is no answer, output -1 -1
The board will be non-empty and rectangular. All values in the board will be either + or 0. All coordinates (input and output) are zero-based. All start positions will be 0, and be on the edge of the board. For example, (0,0) would be the top left corner of any size input.
Snakes may move in any of four directions - up, down, left, or right - one square at a time, but they will never return to a square that they’ve already visited. If a snake enters the board on an edge square, we want to catch it at a different exit square on the board’s edge.
The snake is familiar with the board and will take the route to the nearest reachable exit, in terms of the number of squares it has to move through to get there.
Write a function that takes a rectangular board with only +’s and 0’s, along with a starting point on the edge of the board (given row first, then column), and returns the coordinates of the nearest exit to which it can travel.
If multiple exits are equally close, give the one with the lowest numerical value for the row. If there is still a tie, give the one of those with the lowest numerical value for the column.
If there is no answer, output -1 -1
The board will be non-empty and rectangular. All values in the board will be either + or 0. All coordinates (input and output) are zero-based. All start positions will be 0, and be on the edge of the board. For example, (0,0) would be the top left corner of any size input.
2. Subdomain Visit Count
A website domain "discuss.leetcode.com" consists of various subdomains. At the top level, we have "com", at the next level, we have "leetcode.com" and at the lowest level, "discuss.leetcode.com". When we visit a domain like "discuss.leetcode.com", we will also visit the parent domains "leetcode.com" and "com" implicitly.
A count-paired domain is a domain that has one of the two formats "rep d1.d2.d3" or "rep d1.d2" where rep is the number of visits to the domain and d1.d2.d3 is the domain itself.
- For example, "9001 discuss.leetcode.com" is a count-paired domain that indicates that discuss.leetcode.com was visited 9001 times.
Given an array of count-paired domains cpdomains, return an array of the count-paired domains of each subdomain in the input. You may return the answer in any order.
Example 1:
Input: cpdomains = ["9001 discuss.leetcode.com"] Output: ["9001 leetcode.com","9001 discuss.leetcode.com","9001 com"] Explanation: We only have one website domain: "discuss.leetcode.com". As discussed above, the subdomain "leetcode.com" and "com" will also be visited. So they will all be visited 9001 times.
Example 2:
Input: cpdomains = ["900 google.mail.com", "50 yahoo.com", "1 intel.mail.com", "5 wiki.org"] Output: ["901 mail.com","50 yahoo.com","900 google.mail.com","5 wiki.org","5 org","1 intel.mail.com","951 com"] Explanation: We will visit "google.mail.com" 900 times, "yahoo.com" 50 times, "intel.mail.com" once and "wiki.org" 5 times. For the subdomains, we will visit "mail.com" 900 + 1 = 901 times, "com" 900 + 50 + 1 = 951 times, and "org" 5 times.
Constraints:
- 1 <= cpdomain.length <= 100
- 1 <= cpdomain[i].length <= 100
- cpdomain[i] follows either the "repi d1i.d2i.d3i" format or the "repi d1i.d2i" format.
- repi is an integer in the range [1, 104].
- d1i, d2i, and d3i consist of lowercase English letters.
3. Unique Email Addresses Count
Every valid email consists of a local name and a domain name, separated by the '@' sign. Besides lowercase letters, the email may contain one or more '.' or '+'.
- For example, in "alice@leetcode.com", "alice" is the local name, and "leetcode.com" is the domain name.
If you add periods '.' between some characters in the local name part of an email address, mail sent there will be forwarded to the same address without dots in the local name. Note that this rule does not apply to domain names.
- For example, "alice.z@leetcode.com" and "alicez@leetcode.com" forward to the same email address.
If you add a plus '+' in the local name, everything after the first plus sign will be ignored. This allows certain emails to be filtered. Note that this rule does not apply to domain names.
- For example, "m.y+name@email.com" will be forwarded to "my@email.com".
It is possible to use both of these rules at the same time.
Given an array of strings emails where we send one email to each emails[i], return the number of different addresses that actually receive mails.
Example 1:
Input: emails = ["test.email+alex@leetcode.com","test.e.mail+bob.cathy@leetcode.com","testemail+david@lee.tcode.com"] Output: 2 Explanation: "testemail@leetcode.com" and "testemail@lee.tcode.com" actually receive mails.
Example 2:
Input: emails = ["a@leetcode.com","b@leetcode.com","c@leetcode.com"] Output: 3
Constraints:
- 1 <= emails.length <= 100
- 1 <= emails[i].length <= 100
- emails[i] consist of lowercase English letters, '+', '.' and '@'.
- Each emails[i] contains exactly one '@' character.
- All local and domain names are non-empty.
- Local names do not start with a '+' character.
- Domain names end with the ".com" suffix.
4. Longest Substring Without Repeating Characters
Given a string s, find the length of the longest substring without repeating characters.
Example 1:
Input: s = "abcabcbb" Output: 3 Explanation: The answer is "abc", with the length of 3.
Example 2:
Input: s = "bbbbb" Output: 1 Explanation: The answer is "b", with the length of 1.
Example 3:
Input: s = "pwwkew" Output: 3 Explanation: The answer is "wke", with the length of 3. Notice that the answer must be a substring, "pwke" is a subsequence and not a substring.
Constraints:
- 0 <= s.length <= 5 * 104
- s consists of English letters, digits, symbols and spaces.
5. Spiral Matrix Traversal
Given an m x n matrix, return all elements of the matrix in spiral order.
Example 1:
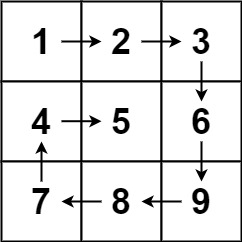
Input: matrix = [[1,2,3],[4,5,6],[7,8,9]] Output: [1,2,3,6,9,8,7,4,5]
Example 2:
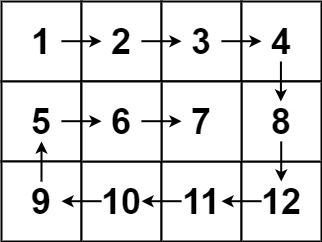
Input: matrix = [[1,2,3,4],[5,6,7,8],[9,10,11,12]] Output: [1,2,3,4,8,12,11,10,9,5,6,7]
Constraints:
- m == matrix.length
- n == matrix[i].length
- 1 <= m, n <= 10
- -100 <= matrix[i][j] <= 100